Class “CameraView”
Object > NativeObject > Widget > CameraView
A widget to preview a Camera
feed.
In order to show a camera preview image the app has to hold the 'camera'
permission.
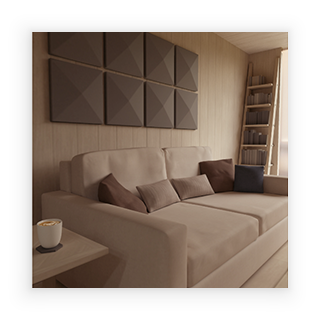
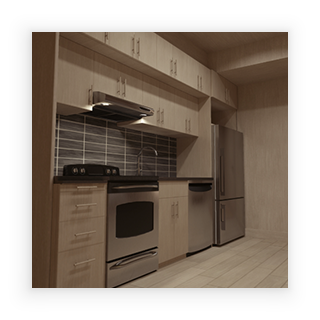
Type: | CameraView extends Widget |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <CameraView/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Not Supported |
Examples
JavaScript
import {CameraView, contentView, device} from 'tabris';
const camera = device.cameras[0];
camera.active = true;
new CameraView({
layoutData: 'stretch',
camera
}).appendTo(contentView);
JSX
import {CameraView, contentView, device} from 'tabris';
const camera = device.cameras[0];
camera.active = true;
contentView.append(
<CameraView stretch camera={camera}/>
);
See also:
TSX Simple example to capture an image [► Run in Playground]
TSX Control Camera
and CameraView
to capture an image [► Run in Playground]
Constructor
new CameraView(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<CameraView> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Properties
camera
The source video feed to display. In order to show a preview the Camera
has to be in an active state. When the Camera
is inactive or no Camera
is assigned the CameraView
shows a blank screen.
It is recommended to deactivate the Camera
when not in use in order to preserve battery life.
Type: | Camera |
Default: | null |
Settable: | Yes |
Change Event: | cameraChanged |
scaleMode
How to scale the camera preview image.
fit
will scale the image proportionally to fit into the view, possible leaving some empty space at the edges. That is, the image will be displayed as large as possible while being fully contained in the view.fill
will scale the image proportionally to fill the entire view, possibly cutting off parts of the image. That is, the image will be displayed as small as possible while covering the entire view.
Type: | 'fit' | 'fill' |
Default: | 'fit' |
Settable: | Yes |
Change Event: | scaleModeChanged |
Change Events
cameraChanged
Fired when the camera property has changed.
EventObject Type: PropertyChangedEvent<CameraView, Camera>
Property | Type | Description |
---|---|---|
value | Camera |
The new value of camera. |
scaleModeChanged
Fired when the scaleMode property has changed.
EventObject Type: PropertyChangedEvent<CameraView, 'fit' | 'fill'>
Property | Type | Description |
---|---|---|
value | 'fit' | 'fill' |
The new value of scaleMode. |