Class “ActionSheet”
Object > NativeObject > Popup > ActionSheet
A pop up dialog that offers a selection. Is automatically disposed when closed.
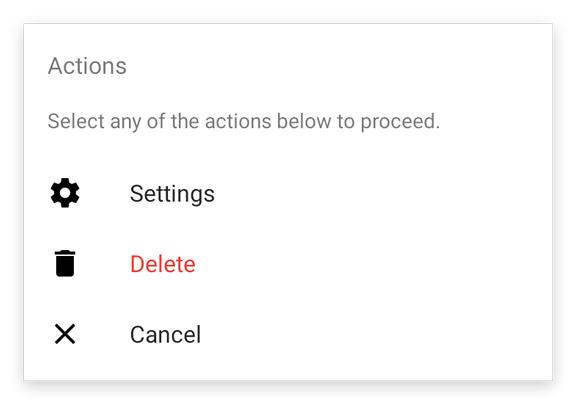
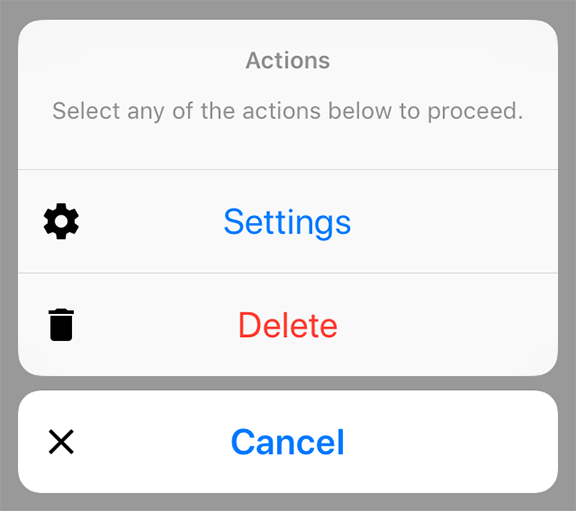
Type: | ActionSheet extends Popup |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <ActionSheet/> Parent Elements: Not supported Child Elements: <ActionSheetItem/> Element content sets: message , actions |
Examples
JavaScript
import {ActionSheet} from 'tabris';
new ActionSheet({
title: 'ActionSheet',
actions: [{title: 'Save'}, {title: 'Delete', style: 'destructive'}]
}).onSelect(({index}) => console.log(`Selected ${index}`))
.open();
See also:
JSX Creating a simple ActionSheet
[► Run in Playground]
Constructor
new ActionSheet(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<ActionSheet> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Static Methods
open(actionSheet)
Makes the given action sheet visible. Meant to be used with inline-JSX. In TypeScript it also casts the given JSX element from any
to an actual ActionSheet.
Parameter | Type | Description |
---|---|---|
actionSheet | ActionSheet |
The action sheet to open |
Returns: ActionSheet
Properties
actions
An array of objects describing the actions to be displayed. The entries may be instances of ActionSheetItem
or plain objects with some or all of the same properties.
Type: | ActionSheetItem[] |
Settable: | Yes |
Change Event: | actionsChanged |
JSX Content Type: | <ActionSheetItem/> |
When using ActionSheet as an JSX element <ActionSheetItem/>
child elements are mapped to this property.
message
A descriptive message for the available actions.
Type: | string |
Settable: | Yes |
Change Event: | messageChanged |
JSX Content Type: | Text |
When using ActionSheet as an JSX element the elements Text content is mapped to this property.
title
The title of the action sheet.
Type: | string |
Settable: | Yes |
Change Event: | titleChanged |
Events
close
Fired when the action sheet was closed.
EventObject Type: ActionSheetCloseEvent<ActionSheet>
Property | Type | Description |
---|---|---|
action | ActionSheetItem | null |
A copy of the selected action as an instance of ActionSheetItem. If no action was selected the value is null . |
index | number | null |
The index of the selected action. If no action was selected the value is null . |
select
Fired when an action was selected. Note: on iOS, tapping outside of an ActionSheet will also fire a select
event. Its parameter will be an index of a button with type cancel
. This happens despite the fact that no button has been pressed.
EventObject Type: ActionSheetSelectEvent<ActionSheet>
Property | Type | Description |
---|---|---|
action | ActionSheetItem |
A copy of the selected action as an instance of ActionSheetItem. |
index | number |
The index of the selected action. |
Change Events
titleChanged
Fired when the title property has changed.
EventObject Type: PropertyChangedEvent<ActionSheet, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of title. |
messageChanged
Fired when the message property has changed.
EventObject Type: PropertyChangedEvent<ActionSheet, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of message. |
actionsChanged
Fired when the actions property has changed.
EventObject Type: PropertyChangedEvent<ActionSheet, Array>
Property | Type | Description |
---|---|---|
value | ActionSheetItem[] |
The new value of actions. |