Class “SearchAction”
Object > NativeObject > Widget > Action > SearchAction
An action that displays a search text field with dynamic proposals when selected. Add a listener on select to implement the action. On input, you may set a list of proposals.
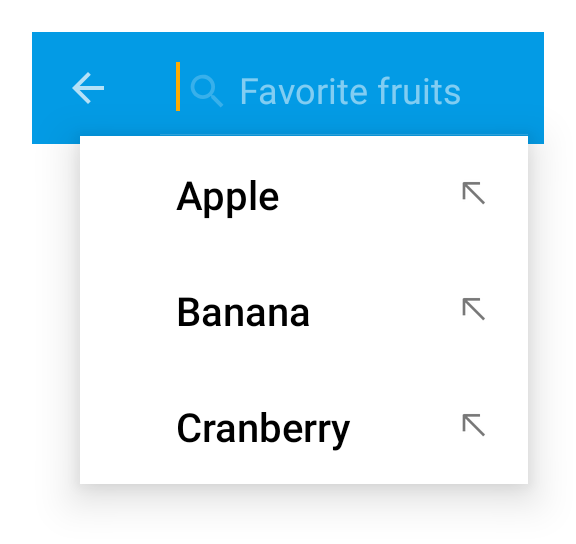
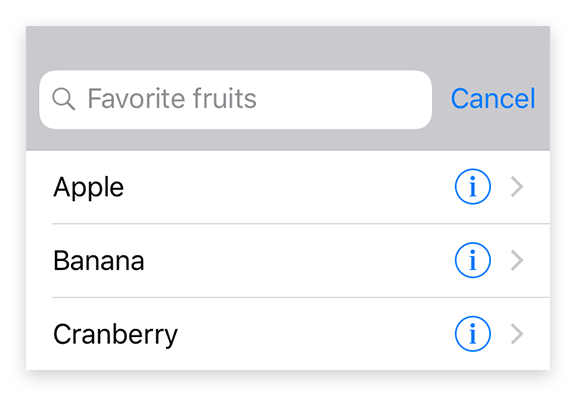
Type: | SearchAction extends Action |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <SearchAction/> Parent Elements: <NavigationView/> Child Elements: Not Supported |
Examples
JavaScript
import {SearchAction, NavigationView, contentView} from 'tabris';
const items = ['apple', 'banana', 'cherry'];
const navigationView = new NavigationView({layoutData: 'stretch'})
.appendTo(contentView);
new SearchAction({title: 'Search', image: 'resources/search.png'})
.onInput(event => items.filter(proposal => proposal.indexOf(event.query) !== -1))
.onAccept(event => console.log(`Showing content for ${event.text}`))
.appendTo(navigationView);
See also:
JSX Creating a SearchAction
[► Run in Playground]
Constructor
new SearchAction(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<SearchAction> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Methods
open()
Invokes the search action, i.e. displays the UI to perform a search.
Returns: undefined
Properties
message
A hint text that is displayed when the search input is empty.
Type: | string |
Settable: | Yes |
Change Event: | messageChanged |
proposals
The list of proposals to display.
Type: | string[] |
Default: | [] |
Settable: | Yes |
Change Event: | proposalsChanged |
text
The text in the search input field.
Type: | string |
Settable: | Yes |
Change Event: | textChanged |
Events
input
Fired when the user inputs text.
EventObject Type: SearchActionInputEvent<SearchAction>
Property | Type | Description |
---|---|---|
text | string |
The new value of text. |
accept
Fired when a text input has been submitted by pressing the keyboard’s search key.
EventObject Type: SearchActionAcceptEvent<SearchAction>
Property | Type | Description |
---|---|---|
text | string |
The current value of text. |
Change Events
proposalsChanged
Fired when the proposals property has changed.
EventObject Type: PropertyChangedEvent<SearchAction, Array>
Property | Type | Description |
---|---|---|
value | string[] |
The new value of proposals. |
textChanged
Fired when the text property has changed.
EventObject Type: PropertyChangedEvent<SearchAction, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of text. |
messageChanged
Fired when the message property has changed.
EventObject Type: PropertyChangedEvent<SearchAction, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of message. |