Class “ImageView”
Object > NativeObject > Widget > ImageView
A widget to display an image.
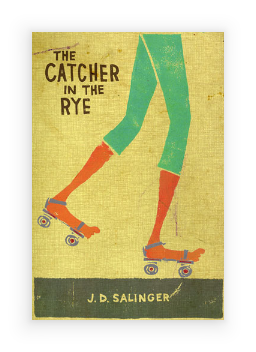
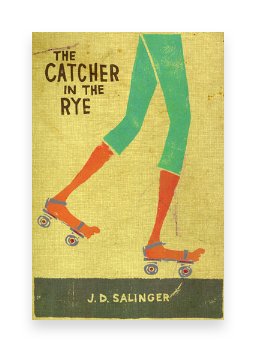
Type: | ImageView extends Widget |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <ImageView/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Not Supported |
Examples
JavaScript
import {ImageView, contentView} from 'tabris';
new ImageView({image: 'resources/image.png'})
.appendTo(contentView);
See also:
JSX Using an ImageView
as a button [► Run in Playground]
JSX Creating ImageView
s with various scale modes [► Run in Playground]
JSX Creating an ImageView
with support for pinch-to-zoom [► Run in Playground]
JSX Creating an ImageView
with a tint color applied [► Run in Playground]
JSX Creating an ImageView
with a load event callback [► Run in Playground]
JSX Using an ImageView
to show a Base64 encoded image [► Run in Playground]
JSX imageview-blob.jsx [► Run in Playground]
TSX imageview-gallery.tsx [► Run in Playground]
JSX imageview-imagebitmap.jsx [► Run in Playground]
JSX imageview-scalemode-auto.jsx [► Run in Playground]
JSX imageview.jsx [► Run in Playground]
Constructor
new ImageView(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<ImageView> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Properties
image
The image to display. Providing the width
and height
attributes on the image will resize it internally. When no dimensions are given the image will be loaded with its original size. Since the full size image might occupy a lot of memory, it’s recommended to provide exact dimensions.
Type: | ImageValue |
Settable: | Yes |
Change Event: | imageChanged |
maxZoomLevel
The highest amount the image can be zoomed in to. Setting the maxZoomLevel
to a level smaller than the current zoomLevel
changes the zoomLevel
to be the same as the new maxZoomLevel
.
Type: | number |
Default: | 3 |
Settable: | Yes |
Change Event: | maxZoomLevelChanged |
minZoomLevel
The lowest amount the image can be zoomed out to. Setting the minZoomLevel
to a level larger than the current zoomLevel
changes the zoomLevel
to be the same as the new minZoomLevel
.
Type: | number |
Default: | 1 |
Settable: | Yes |
Change Event: | minZoomLevelChanged |
scaleMode
How to scale the image.
fit
will scale the image proportionally to fit into the view, possible leaving some empty space at the edges. That is, the image will be displayed as large as possible while being fully contained in the view.fill
will scale the image proportionally to fill the entire view, possibly cutting off parts of the image. That is, the image will be displayed as small as possible while covering the entire view.auto
will scale down the image to fit into the view if it is too large, but it won’t scale up a smaller image.stretch
will resize the image to the actual bounds of the image view.none
will not resize the image at all. The image will be displayed in its original size.
Type: | 'auto' | 'fit' | 'fill' | 'stretch' | 'none' |
Default: | 'auto' |
Settable: | Yes |
Change Event: | scaleModeChanged |
See also:
JSX imageview-scalemode-auto.jsx [► Run in Playground]
JSX imageview-scalemode.jsx [► Run in Playground]
tintColor
A color to change the image appearance. All opaque parts of the image will be tinted with the given color. Set to initial
to remove the effect.
Type: | ColorValue |
Settable: | Yes |
Change Event: | tintColorChanged |
See also:
JSX imageview-tintcolor.jsx [► Run in Playground]
zoomEnabled
Enables the pinch-to-zoom gesture on the ImageView
and makes the properties zoomLevel
, minZoomLevel
and maxZoomLevel
available. Setting zoomEnabled
to false
also resets the zoomLevel
, minZoomLevel
, maxZoomLevel
to their respective defaults.
Type: | boolean |
Default: | false |
Settable: | Yes |
Change Event: | zoomEnabledChanged |
zoomLevel
The amount that the image is zoomed in or out. The default position without any zooming has the value 1.0.
Type: | number |
Default: | 1 |
Settable: | Yes |
Change Event: | zoomLevelChanged |
Events
load
Fired when the image loading has finished.
EventObject Type: ImageViewLoadEvent<ImageView>
Property | Type | Description |
---|---|---|
error | boolean |
Contains the final status of the loading process |
See also:
JSX imageview-load.jsx [► Run in Playground]
zoom
Fired when the user zooms the image in or out. The zoom
event indicates a change to the zoomLevel
property.
EventObject Type: ImageViewZoomEvent<ImageView>
Property | Type | Description |
---|---|---|
zoomLevel | number |
The new value of zoomLevel. |
See also:
JSX imageview-zoom.jsx [► Run in Playground]
Change Events
imageChanged
Fired when the image property has changed.
EventObject Type: PropertyChangedEvent<ImageView, ImageValue>
Property | Type | Description |
---|---|---|
value | ImageValue |
The new value of image. |
scaleModeChanged
Fired when the scaleMode property has changed.
EventObject Type: PropertyChangedEvent<ImageView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of scaleMode. |
tintColorChanged
Fired when the tintColor property has changed.
EventObject Type: PropertyChangedEvent<ImageView, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of tintColor. |
zoomEnabledChanged
Fired when the zoomEnabled property has changed.
EventObject Type: PropertyChangedEvent<ImageView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of zoomEnabled. |
zoomLevelChanged
Fired when the zoomLevel property has changed.
EventObject Type: PropertyChangedEvent<ImageView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of zoomLevel. |
minZoomLevelChanged
Fired when the minZoomLevel property has changed.
EventObject Type: PropertyChangedEvent<ImageView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of minZoomLevel. |
maxZoomLevelChanged
Fired when the maxZoomLevel property has changed.
EventObject Type: PropertyChangedEvent<ImageView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of maxZoomLevel. |