Class “TextInput”
Object > NativeObject > Widget > TextInput
A widget that allows to enter text.
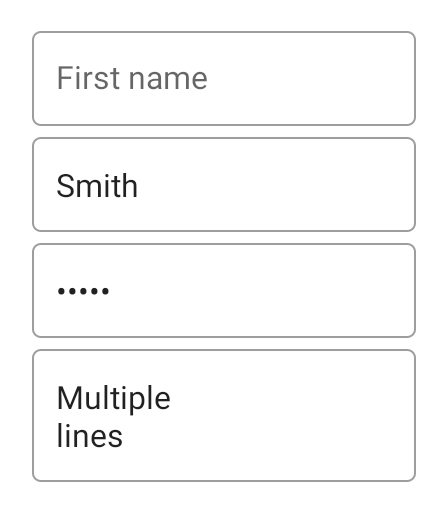
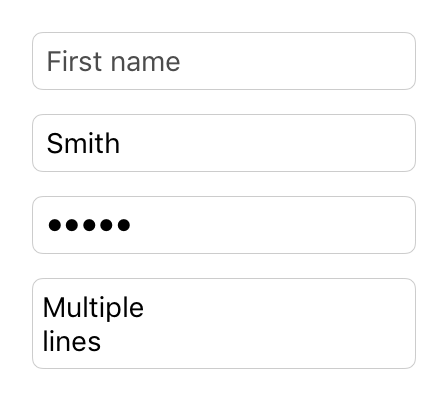
Type: | TextInput extends Widget |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <TextInput/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Not Supported Element content sets: text |
Examples
JavaScript
import {TextInput, contentView} from 'tabris';
new TextInput({
left: 16, right: 16,
message: 'Name'
}).onInput(({text}) => console.log(`Text changed to ${text}`))
.appendTo(contentView);
See also:
JSX Creating a simple TextInput
[► Run in Playground]
JS Handling selection on a TextInput
[► Run in Playground]
JSX Handling focus changes on a TextInput
[► Run in Playground]
JS Creating TextInputs
with various enter key types [► Run in Playground]
JS Creating TextInputs
with various keyboards [► Run in Playground]
JS Showing password in clear text on a TextInput
[► Run in Playground]
JSX A form using TextInput
and other input controls [► Run in Playground]
TSX textinput-focus.tsx [► Run in Playground]
TS textinput-keyboard.ts [► Run in Playground]
TSX textinput-style.tsx [► Run in Playground]
TS textinput-validation.ts [► Run in Playground]
Constructor
new TextInput(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<TextInput> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Properties
alignment
The horizontal alignment of the text.
Type: | 'left' | 'right' | 'centerX' |
Default: | 'left' |
Settable: | Yes |
Change Event: | alignmentChanged |
autoCapitalize
Control how text input is capitalized.
none
- Do not change any text inputsentence
- Capitalize the first word of a sentenceword
- Capitalize every wordall
- Capitalize every letter
The boolean value false
maps to none
whereas true
is equal to all
.
Type: | true | false | none | sentence | word | all |
Default: | false |
Settable: | Yes |
Change Event: | autoCapitalizeChanged |
autoCorrect
Enables the spell checker and auto-correction feature.
Type: | boolean |
Default: | false |
Settable: | Yes |
Change Event: | autoCorrectChanged |
borderColor
The color of the border of the TextInput. On iOS this is a rectangular border around the TextInput, on Android it is a single line below the TextInput.
Type: | ColorValue |
Settable: | Yes |
Change Event: | borderColorChanged |
cursorColor
The color of the cursor in the TextInput
.
Type: | ColorValue |
Settable: | Yes |
Change Event: | cursorColorChanged |
editable
Whether the text can be edited or not.
Type: | boolean |
Default: | true |
Settable: | Yes |
Change Event: | editableChanged |
enterKeyType
Label or icon to display on the keyboard ‘confirmation’ key. The key press can be captured via the accept
event. Setting an enterKeyType
other than default
will change the key behavior to not close the keyboard automatically. The developer is able close the keyboard by removing the focus from the TextInput
.
Type: | 'default' | 'done' | 'next' | 'send' | 'search' | 'go' |
Default: | 'default' |
Settable: | Yes |
Change Event: | enterKeyTypeChanged |
See also:
JS textinput-enterkeytype.js [► Run in Playground]
floatMessage
Android
Should the hint message float above the TextInput when focus is gained.
Type: | boolean |
Default: | true |
Settable: | Yes |
Change Event: | floatMessageChanged |
focused
Reflects whether this widget has the keyboard focus. Setting this property to true
will focus the widget and open the virtual keyboard, setting it to false
will remove the focus and hide the virtual keyboard.
Type: | boolean |
Default: | false |
Settable: | Yes |
Change Event: | focusedChanged |
font
The font used for the text.
Type: | FontValue |
Settable: | Yes |
Change Event: | fontChanged |
keepFocus
When true
the TextInput
will keep its focus, even when tapped outside of the widget bounds.
Type: | boolean |
Default: | false |
Settable: | Yes |
Change Event: | keepFocusChanged |
keyboard
Selects the keyboard type to use for editing this widget. Has no effect when type
is set to multiline
.
Type: | 'ascii' | 'decimal' | 'email' | 'number' | 'numbersAndPunctuation' | 'phone' | 'url' | 'default' |
Default: | 'default' |
Settable: | Yes |
Change Event: | keyboardChanged |
See also:
JS textinput-keyboard.js [► Run in Playground]
TS textinput-keyboard.ts [► Run in Playground]
keyboardAppearanceMode
Android
Allows to control when to show the virtual keyboard.
-
'never'
- The keyboard is never shown when focus is gained. -
'ontouch'
- The keyboard is not shown when thefocused
property is set totrue
programmatically. Only the blinking cursor will be shown. Touching theTextInput
will show the keyboard. -
'onfocus'
- The keyboard is always shown when theTextInput
gains focus.
Type: | 'never' |
Default: | 'onfocus' |
Settable: | Yes |
Change Event: | keyboardAppearanceModeChanged |
maxChars
The maximum number of characters that can be entered into a TextInput
.
Type: | number |
Default: | null |
Settable: | Yes |
Change Event: | maxCharsChanged |
message
A hint text that is displayed when the input field is empty. Does not apply on iOS when type
is set to multiline
.
Type: | string |
Settable: | Yes |
Change Event: | messageChanged |
messageColor
Color of the message
text.
Type: | ColorValue |
Settable: | Yes |
Change Event: | messageColorChanged |
revealPassword
Makes the text visible when the TextInput
has the type password
.
Type: | boolean |
Settable: | Yes |
Change Event: | revealPasswordChanged |
See also:
JS textinput-revealpassword.js [► Run in Playground]
selection
The selection
is a two element number array representing the text selections start and end position. The native platform usually shows selection handles so that the selection can be changed by the user. A selection
array where both numbers are the same represent a single cursor at the given position. The selection start is the index of the first character where as the end is the index of the last character + 1. E.g. to select the word “ok” the selection would be [0, 2]
.
To make a selection visible the TextInput
has to be in focus. Consequently the selection is preserved when the focus is lost and regained. When the user gives the TextInput
focus by tapping on it, the selection is changed to represent his touch position.
Getting the selection
upon user interaction (e.g. a button press) the focus would be lost and possibly the selection
altered due to user interaction. In such a scenario it is recommended to set the keepFocus
property to true
.
Type: | number[] |
Settable: | Yes |
Change Event: | selectionChanged |
See also:
JS textinput-selection.js [► Run in Playground]
style
Android
The visual appearance of the text widget.
With the style
outline, fill or underline the message hint will float above the TextInput
on Android. This behavior can be controlled with the property floatMessage
. The style
none will remove any background visualization, allowing to create a custom background.
Type: | 'default' | 'outline' | 'fill' | 'underline' | 'none' |
Default: | 'default' |
Settable: | By Constructor or JSX |
Change Event: | Not supported |
This property can only be set via constructor or JSX. Once set, it cannot change anymore.
See also:
TSX textinput-style.tsx [► Run in Playground]
text
The text in the input field.
Type: | string |
Settable: | Yes |
Change Event: | textChanged |
JSX Content Type: | Text |
When using TextInput as an JSX element the elements Text content is mapped to this property.
textColor
The color of the text.
Type: | ColorValue |
Settable: | Yes |
Change Event: | textColorChanged |
type
The type of the text widget.
Type: | 'default' | 'password' | 'search' | 'multiline' |
Default: | 'default' |
Settable: | By Constructor or JSX |
Change Event: | Not supported |
This property can only be set via constructor or JSX. Once set, it cannot change anymore.
Events
accept
Fired when a text input has been finished by pressing the keyboard’s Enter key. The label of this key may vary depending on the platform and locale.
EventObject Type: TextInputAcceptEvent<TextInput>
Property | Type | Description |
---|---|---|
text | string |
The current value of text. |
select
The select
event is fired when the user alters the text selection
. Either by dragging the selection handles of a text selection, by moving the cursor inside the text or by typing which also advances the cursor.
The event also fires when the user taps inside a TextInput
since this involves to set the cursor to the tapped position.
EventObject Type: TextInputSelectEvent<TextInput>
Property | Type | Description |
---|---|---|
selection | number[] |
The current selection as a two element number array of start and end position. |
blur
Fired when the widget lost focus.
EventObject Type: EventObject<TextInput>
This event has no additional parameter.
focus
Fired when the widget gains focus.
EventObject Type: EventObject<TextInput>
This event has no additional parameter.
See also:
TSX textinput-focus.tsx [► Run in Playground]
input
Fired when the text was changed by the user.
EventObject Type: TextInputInputEvent<TextInput>
Property | Type | Description |
---|---|---|
text | string |
The new value of text. |
beforeTextChange
Fired when the text value is about to change due to a user action.
EventObject Type: TextInputBeforeTextChangeEvent<TextInput>
Property | Type | Description |
---|---|---|
newValue | string |
The text after the change, i.e. the future text value if preventDefault is not called. |
oldValue | string |
The text before the change, i.e. the current text value. |
preventDefault | Function |
Call to intercept the change. |
Change Events
textChanged
Fired when the text property has changed.
EventObject Type: PropertyChangedEvent<TextInput, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of text. |
textColorChanged
Fired when the textColor property has changed.
EventObject Type: PropertyChangedEvent<TextInput, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of textColor. |
messageChanged
Fired when the message property has changed.
EventObject Type: PropertyChangedEvent<TextInput, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of message. |
messageColorChanged
Fired when the messageColor property has changed.
EventObject Type: PropertyChangedEvent<TextInput, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of messageColor. |
editableChanged
Fired when the editable property has changed.
EventObject Type: PropertyChangedEvent<TextInput, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of editable. |
maxCharsChanged
Fired when the maxChars property has changed.
EventObject Type: PropertyChangedEvent<TextInput, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of maxChars. |
floatMessageChanged
Fired when the floatMessage property has changed.
EventObject Type: PropertyChangedEvent<TextInput, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of floatMessage. |
alignmentChanged
Fired when the alignment property has changed.
EventObject Type: PropertyChangedEvent<TextInput, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of alignment. |
autoCorrectChanged
Fired when the autoCorrect property has changed.
EventObject Type: PropertyChangedEvent<TextInput, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of autoCorrect. |
autoCapitalizeChanged
Fired when the autoCapitalize property has changed.
EventObject Type: PropertyChangedEvent<TextInput, any>
Property | Type | Description |
---|---|---|
value | any |
The new value of autoCapitalize. |
keyboardChanged
Fired when the keyboard property has changed.
EventObject Type: PropertyChangedEvent<TextInput, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of keyboard. |
enterKeyTypeChanged
Fired when the enterKeyType property has changed.
EventObject Type: PropertyChangedEvent<TextInput, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of enterKeyType. |
focusedChanged
Fired when the focused property has changed.
EventObject Type: PropertyChangedEvent<TextInput, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of focused. |
keepFocusChanged
Fired when the keepFocus property has changed.
EventObject Type: PropertyChangedEvent<TextInput, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of keepFocus. |
borderColorChanged
Fired when the borderColor property has changed.
EventObject Type: PropertyChangedEvent<TextInput, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of borderColor. |
revealPasswordChanged
Fired when the revealPassword property has changed.
EventObject Type: PropertyChangedEvent<TextInput, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of revealPassword. |
cursorColorChanged
Fired when the cursorColor property has changed.
EventObject Type: PropertyChangedEvent<TextInput, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of cursorColor. |
selectionChanged
Fired when the selection property has changed.
EventObject Type: PropertyChangedEvent<TextInput, Array>
Property | Type | Description |
---|---|---|
value | number[] |
The new value of selection. |
fontChanged
Fired when the font property has changed.
EventObject Type: PropertyChangedEvent<TextInput, FontValue>
Property | Type | Description |
---|---|---|
value | FontValue |
The new value of font. |
keyboardAppearanceModeChanged
Fired when the keyboardAppearanceMode property has changed.
EventObject Type: PropertyChangedEvent<TextInput, 'never'
| 'ontouch'
| 'onfocus'>
Property | Type | Description |
---|---|---|
value | 'never' |
The new value of keyboardAppearanceMode. |