Class “WebView”
Object > NativeObject > Widget > WebView
A widget that can display a web page. Since this widget requires a lot of resources it’s recommended to have no more than one instance at a time.
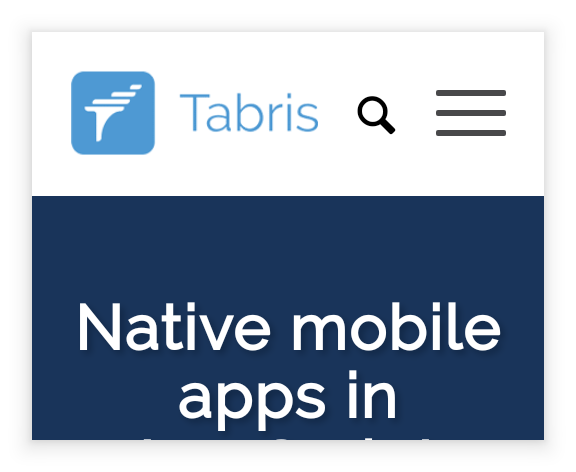
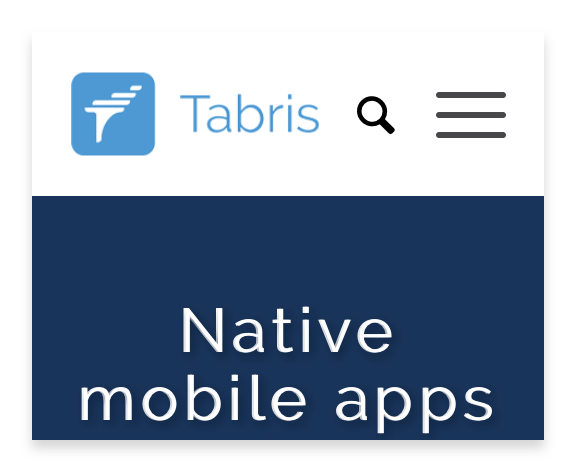
Type: | WebView extends Widget |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <WebView/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Not Supported |
Examples
JavaScript
import {WebView, contentView} from 'tabris';
new WebView({
left: 0, right: 0, top: 0, bottom: 0,
url: 'https://tabris.com'
}).appendTo(contentView);
See also:
JSX Creating a simple WebView
[► Run in Playground]
JS Creating browser-like navigation buttons in a WebView
[► Run in Playground]
JS Demonstrating web messaging in a WebView
[► Run in Playground]
Web Messaging on Wikipedia
Constructor
new WebView(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<WebView> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Methods
goBack()
Navigate the WebView
to the previous page if possible.
Returns: undefined
goForward()
Navigate the WebView
to the next page if possible.
Returns: undefined
postMessage(message, targetOrigin)
Posts a web message to the underlying window
object of the WebView. The website in the WebView
can register for the message in the following fashion: window.addEventListener('message', callbackFunction)
. For more information see Window.postMessage()
API.
Parameter | Type | Description |
---|---|---|
message | string |
The message to send. Supports only strings. |
targetOrigin | string |
The URL of the page that receives the message. The message is only sent if the current document URL has the same scheme, domain and path. Use * to send to any URL. |
Returns: this
Properties
canGoBack
Whether there is a previous to navigated to via goBack()
.
Type: | boolean |
Settable: | No |
Change Event: | canGoBackChanged |
canGoForward
Whether there is a next page to navigate to via goForward()
.
Type: | boolean |
Settable: | No |
Change Event: | canGoForwardChanged |
html
A complete HTML document to display. Always returns the last set value.
Note: htmlChanged
event will not be fired on iOS when a page is using history.pushState()
to navigate between pages.
Type: | string |
Settable: | Yes |
Change Event: | htmlChanged |
initScript
JavaScript code to be executed before page begins loading.
Type: | string |
Settable: | By Constructor or JSX |
Change Event: | Not supported |
This property can only be set via constructor or JSX. Once set, it cannot change anymore.
url
The URL of the web page to display. Relative URLs are resolved relative to ‘package.json’. Returns empty string when content from html property is displayed.
Note: urlChanged
event will not be fired on iOS when a page is using history.pushState()
to navigate between pages.
Type: | string |
Settable: | Yes |
Change Event: | urlChanged |
Events
navigate
Fired when the WebView is about to navigate to a new URL.
Note: This event will not be fired on iOS when a page is using history.pushState()
to navigate between pages.
EventObject Type: WebViewNavigateEvent<WebView>
Property | Type | Description |
---|---|---|
preventDefault | Function |
Call to intercept the navigation. Not possible when the event is only an anchor navigation |
url | string |
The new URL the WebView is about to navigate to. |
load
Fired when the url has been loaded.
Note: This event will not be fired on iOS when a page is using history.pushState()
to navigate between pages.
EventObject Type: EventObject<WebView>
This event has no additional parameter.
download
Android
Fired when the WebView requests a download. The download event provides the properties url
, mimeType
, contentLength
and contentDisposition
. Supported only on Android.
EventObject Type: WebViewDownloadEvent<WebView>
Property | Type | Description |
---|---|---|
contentDisposition | string |
Indicates whether the download is expected to be displayed inline or to be downloaded as an attachment. |
contentLength | number |
The size of the downloaded entity body. |
mimeType | string |
The mime type of the resource to be downloaded. |
url | string |
The URL of the resource to be downloaded. |
message
Fired when a web message has been sent via window.parent.postMessage(message, targetOrigin)
from inside the WebView
.
EventObject Type: WebViewMessageEvent<WebView>
Property | Type | Description |
---|---|---|
data | string |
The sent message. |
Change Events
urlChanged
Fired when the url property has changed.
EventObject Type: PropertyChangedEvent<WebView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of url. |
htmlChanged
Fired when the html property has changed.
EventObject Type: PropertyChangedEvent<WebView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of html. |
canGoForwardChanged
Fired when the canGoForward property has changed.
EventObject Type: PropertyChangedEvent<WebView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of canGoForward. |
canGoBackChanged
Fired when the canGoBack property has changed.
EventObject Type: PropertyChangedEvent<WebView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of canGoBack. |