Class “NavigationView”
Object > NativeObject > Widget > Composite > NavigationView
A widget that displays a stack of pages with a toolbar that allows to navigate back. The toolbar also displays the current page’s title and the highest priority actions that are added to the NavigationView. Only children of type Page
, Action
and SearchAction
are supported. Since the NavigationView does not compute its own size, the width and height must be defined by the respective layout properties (e.g. either width
or left
and right
must be specified).
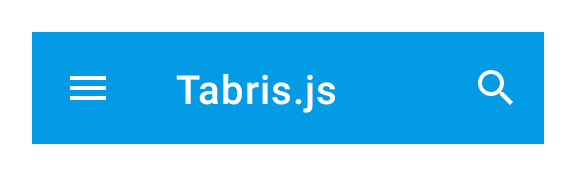
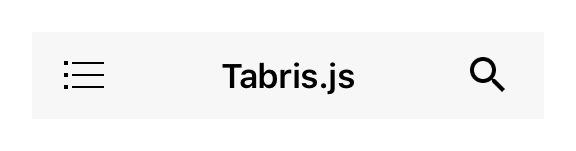
Type: | NavigationView<PageType, ActionType> |
Generics: | PageType: The common widget class of the pages this NavigationView can contain. Must be a subclass of Page and defaults to Page .ActionType: The common widget class of the actions this NavigationView can contain. Must be a subclass of Action and defaults to Action . |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <NavigationView/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: <Page/> , <Action/> , <SearchAction/> |
Examples
JavaScript
import {NavigationView, Page, contentView} from 'tabris';
new NavigationView({layoutData: 'stretch'})
.append(new Page({title: 'Albums'}))
.appendTo(contentView);
See also:
JSX Creating a simple NavigationView
with pages [► Run in Playground]
JSX Demonstrating a NavigationView
with various interactive properties [► Run in Playground]
JS Using multiple NavigationViews
in TabFolder
Tabs
[► Run in Playground]
JSX navigationview-action-placement.jsx [► Run in Playground]
JSX navigationview-action.jsx [► Run in Playground]
JSX navigationview-searchaction.jsx [► Run in Playground]
Constructor
new NavigationView(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<NavigationView> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Methods
pages(selector?)
Returns the ordered list of pages on the page stack, with the bottommost page as the first and the topmost page as the last element. Same as children(), but only returns children that are of type Page
.
Parameter | Type | Description |
---|---|---|
selector | Selector |
A selector expression or a predicate function to filter the results. Optional. |
Returns: WidgetCollection
Properties
actionColor
The color used for action icons.
Type: | ColorValue |
Settable: | Yes |
Change Event: | actionColorChanged |
actionTextColor
Android
The color used for action texts. Only applied on Android, IOS uses the actionColor
to colorize the action text.
Type: | ColorValue |
Settable: | Yes |
Change Event: | actionTextColorChanged |
drawerActionVisible
Whether to display the so-called “Burger menu” to open the drawer.
Type: | boolean |
Default: | false |
Settable: | Yes |
Change Event: | drawerActionVisibleChanged |
pageAnimation
Controls what animation to use when animating a page transition.
Type: | 'default' | 'none' |
Default: | 'default' |
Settable: | Yes |
Change Event: | pageAnimationChanged |
titleTextColor
The text color used for page titles.
Type: | ColorValue |
Settable: | Yes |
Change Event: | titleTextColorChanged |
toolbarColor
The background color of the toolbar.
Type: | ColorValue |
Settable: | Yes |
Change Event: | toolbarColorChanged |
toolbarHeight
The height of the toolbar. Is 0 if not visible.
Type: | number |
Settable: | Yes |
Change Event: | toolbarHeightChanged |
toolbarVisible
Whether the toolbar is visible.
Type: | boolean |
Default: | true |
Settable: | Yes |
Change Event: | toolbarVisibleChanged |
Change Events
drawerActionVisibleChanged
Fired when the drawerActionVisible property has changed.
EventObject Type: PropertyChangedEvent<NavigationView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of drawerActionVisible. |
toolbarVisibleChanged
Fired when the toolbarVisible property has changed.
EventObject Type: PropertyChangedEvent<NavigationView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of toolbarVisible. |
toolbarColorChanged
Fired when the toolbarColor property has changed.
EventObject Type: PropertyChangedEvent<NavigationView, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of toolbarColor. |
toolbarHeightChanged
Fired when the height of the toolbar changes, e.g. if it changes visibility.
EventObject Type: PropertyChangedEvent<NavigationView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of toolbarHeight. |
titleTextColorChanged
Fired when the titleTextColor property has changed.
EventObject Type: PropertyChangedEvent<NavigationView, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of titleTextColor. |
actionColorChanged
Fired when the actionColor property has changed.
EventObject Type: PropertyChangedEvent<NavigationView, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of actionColor. |
actionTextColorChanged
Fired when the actionTextColor property has changed.
EventObject Type: PropertyChangedEvent<NavigationView, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of actionTextColor. |
pageAnimationChanged
Fired when the pageAnimation property has changed.
EventObject Type: PropertyChangedEvent<NavigationView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of pageAnimation. |