Class “Canvas”
Object > NativeObject > Widget > Composite > Canvas
Canvas is a widget that can be used to draw graphics using a canvas context.
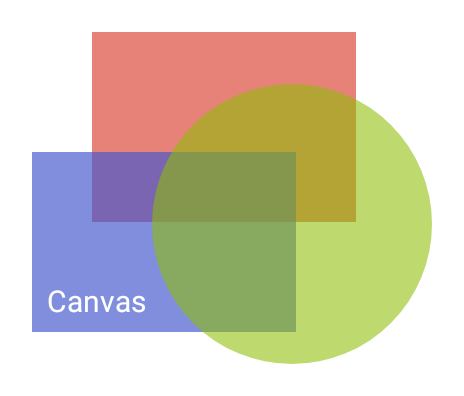
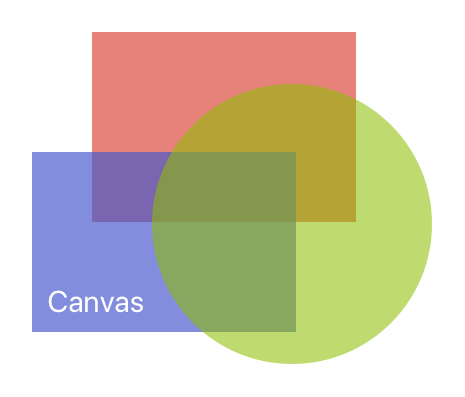
Type: | Canvas extends Composite |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <Canvas/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Any standalone widget element |
Examples
JavaScript
import {Canvas, contentView} from 'tabris';
new Canvas({layoutData: 'stretch'})
.onResize(({target: canvas, width, height}) => {
const context = canvas.getContext('2d', width, height);
context.moveTo(0, 0);
// ...
}).appendTo(contentView);
See also:
JSX Creating a Canvas
with simple shapes
JSX Creating a Canvas
and working with ImageData
JSX How to show text on a Canvas
JSX How to use animations on a Canvas
JSX canvas-arc.jsx
JSX canvas-imagebitmap.jsx
JSX canvas-to-image.jsx
Constructor
new Canvas(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<Canvas> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Methods
getContext(contextType, width, height)
Returns the drawing context with the given size.
Parameter | Type | Description |
---|---|---|
contextType | string |
The context identifier. Only "2d" is supported. |
width | number |
the width of the canvas context to create |
height | number |
the height of the canvas context to create |
Returns: CanvasContext
toBlob(callback, mimeType?, quality?)
Creates a Blob object representing the image contained in the canvas. This is a non-blocking operation.
Parameter | Type | Description |
---|---|---|
callback | (blob) => void |
Callback that will be called with the resulting blob. |
mimeType | 'image/png' |
The expected image format. If mimeType is not specified or invalid, the image type is image/png . On iOS the type image/webp is not supported. A png will be returned instead. Optional. |
quality | number |
A Number between 0 and 1 specifying the image quality. A lower number results in a smaller file size at the same resolution. If quality is not specified or invalid the value 0.92 is for image/jpeg and 0.80 for image/webp respectively. The value has no effect for image/png . Optional. |
Returns: undefined