Class “ScrollView”
Object > NativeObject > Widget > Composite > ScrollView
A composite that allows its content to overflow either vertically (default) or horizontally. Since the ScrollView does not compute its own size, the width and height must be defined by the respective layout properties (e.g. either width
or left
and right
must be specified).
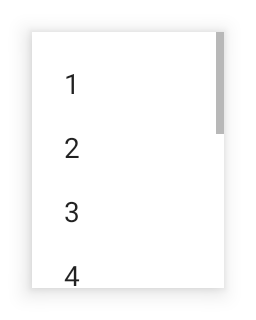
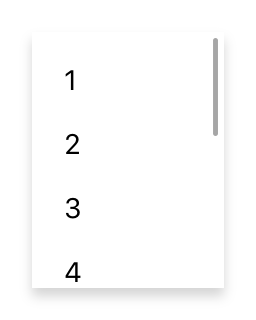
Type: | ScrollView extends Composite |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <ScrollView/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Any standalone widget element |
Examples
JavaScript
import {ScrollView, TextView, contentView} from 'tabris';
const scrollView = new ScrollView({layoutData: 'stretch'})
.appendTo(contentView);
new TextView({text: 'Scrollable content'})
.appendTo(scrollView);
See also:
JSX Creating a simple ScrollView
JSX How to implement parallax scrolling with a ScrollView
Constructor
new ScrollView(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<ScrollView> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Methods
scrollToX(offset, options?)
Scrolls to the given horizontal offset. Give {animate: false}
as the second parameter to suppress the animation.
Parameter | Type | Description |
---|---|---|
offset | number |
The offset to scroll to in dip. |
options | { |
An additional parameter object to control the animation. Optional. |
Returns: this
scrollToY(offset, options?)
Scrolls to the given vertical offset. Give {animate: false}
as the second parameter to suppress the animation.
Parameter | Type | Description |
---|---|---|
offset | number |
The offset to scroll to in dip. |
options | { |
An parameter object to control the animation. Optional. |
Returns: this
Properties
direction
Specifies the scrolling direction of the scroll composite.
Type: | 'vertical' | 'horizontal' |
Default: | 'vertical' |
Settable: | By Constructor or JSX |
Change Event: | Not supported |
This property can only be set via constructor or JSX. Once set, it cannot change anymore.
offsetX
The horizontal scrolling position in dip.
Type: | number |
Settable: | No |
Change Event: | offsetXChanged |
offsetY
The vertical scrolling position in dip.
Type: | number |
Settable: | No |
Change Event: | offsetYChanged |
scrollXState
The scroll state of the ScrollView
in horizontal direction. The following states are supported:
rest
- no scrollingdrag
the user moves theScrollView
content with his fingerscroll
the user has flinged the content with his finger or theScrollView
is scrolling programmatically
Type: | 'rest' | 'drag' | 'scroll' |
Default: | 'rest' |
Settable: | No |
Change Event: | scrollXStateChanged |
scrollYState
The scroll state of the ScrollView
in vertical direction. The following states are supported:
rest
- no scrollingdrag
the user moves theScrollView
content with his fingerscroll
the user has flinged theScrollView
content with his finger or theScrollView
is scrolling programmatically
Type: | 'rest' | 'drag' | 'scroll' |
Default: | 'rest' |
Settable: | No |
Change Event: | scrollYStateChanged |
scrollbarVisible
Allows to show or hide scroll bar for current direction.
Type: | boolean |
Default: | true |
Settable: | Yes |
Change Event: | scrollbarVisibleChanged |
Events
scrollX
Fired while scrolling horizontally.
EventObject Type: ScrollViewScrollEvent<ScrollView>
Property | Type | Description |
---|---|---|
offset | number |
Indicates the current horizontal scrolling position. |
scrollY
Fired while scrolling vertically.
EventObject Type: ScrollViewScrollEvent<ScrollView>
Property | Type | Description |
---|---|---|
offset | number |
Indicates the current vertical scrolling position. |
Change Events
offsetXChanged
Fired when the offsetX property has changed.
EventObject Type: PropertyChangedEvent<ScrollView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of offsetX. |
offsetYChanged
Fired when the offsetY property has changed.
EventObject Type: PropertyChangedEvent<ScrollView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of offsetY. |
scrollbarVisibleChanged
Fired when the scrollbarVisible property has changed.
EventObject Type: PropertyChangedEvent<ScrollView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of scrollbarVisible. |
scrollXStateChanged
Fired when the scrollXState property has changed.
EventObject Type: PropertyChangedEvent<ScrollView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of scrollXState. |
scrollYStateChanged
Fired when the scrollYState property has changed.
EventObject Type: PropertyChangedEvent<ScrollView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of scrollYState. |