Class “TextView”
Object > NativeObject > Widget > TextView
A widget to display a text. For images, use ImageView.
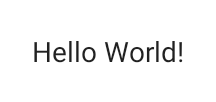
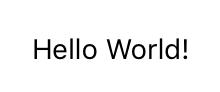
Type: | TextView extends Widget |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <TextView/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Not Supported Element content sets: text |
Examples
JavaScript
import {TextView, contentView} from 'tabris';
new TextView({
left: 16, right: 16,
text: 'Content'
}).appendTo(contentView);
See also:
JSX Creating a simple TextView
JSX Creating a TextView
with support for markup
JSX Creating a TextView
with support for text links
JSX Demonstrating various line spacing values on a TextView
JS Creating a TextView
with an external font
JS textview-font-bundled.js
JS textview-linespacing.js
Constructor
new TextView(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<TextView> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Properties
alignment
The horizontal alignment of the text.
Type: | 'left' | 'right' | 'centerX' |
Default: | 'left' |
Settable: | Yes |
Change Event: | alignmentChanged |
font
The font used for the text.
Type: | FontValue |
Settable: | Yes |
Change Event: | fontChanged |
See also:
JS textview-font-bundled.js
JS textview-font-external.js
lineSpacing
The amount of space between each line of text. The lineSpacing
property is a factor with a default value of 1.0
.
Type: | number |
Default: | 1.0 |
Settable: | Yes |
Change Event: | lineSpacingChanged |
See also:
markupEnabled
Allows for a subset of HTML tags in the text. Supported tags are: a
, del
, ins
, b
, i
, strong
, em
, big
, small
, br
.
All tags except <br/>
support the attributes “font” and “textColor” with the same values as the TextView
itself. IOS does not support to colorize links (<a>
) with the textColor
attribute.
All tags must be closed (e.g. use <br/>
instead of <br>
). Nesting tags is not supported on iOS. A platform might allow to use additional tags but cross-platform compatibility is only guaranteed for the tags listed above.
When the text is given as the content of a <TextView>
JSX element, markupEnabled
will parse the text more like HTML, i.e. consecutive white spaces will be merged.
Type: | boolean |
Settable: | Yes |
Change Event: | markupEnabledChanged |
See also:
JSX textview-markupenabled.jsx
maxLines
Limit the number of lines to be displayed to the given maximum. null
disables this limit.
Type: | number | null |
Default: | null |
Settable: | Yes |
Change Event: | maxLinesChanged |
selectable
Android
Whether the text can be selected or not.
Type: | boolean |
Settable: | Yes |
Change Event: | selectableChanged |
text
The text to display.
Type: | string |
Settable: | Yes |
Change Event: | textChanged |
JSX Content Type: | Text |
When using TextView as an JSX element the elements Text content is mapped to this property.
textColor
The color of the text.
Type: | ColorValue |
Settable: | Yes |
Change Event: | textColorChanged |
Events
tapLink
Fires when the user clicks on a link in an html text. Requires to set markupEnabled
to true and to provide a text containing an anchor (<a>
) with an href
attribute. Eg. textView.text = 'Website: <a href="http://example.com>example.com</a>'
. The event object contains a property url
which provides the anchors href
url.
EventObject Type: TextViewTapLinkEvent<TextView>
Property | Type | Description |
---|---|---|
url | string |
The url referenced by the href attribute of the anchor. |
Change Events
alignmentChanged
Fired when the alignment property has changed.
EventObject Type: PropertyChangedEvent<TextView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of alignment. |
selectableChanged
Fired when the selectable property has changed.
EventObject Type: PropertyChangedEvent<TextView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of selectable. |
markupEnabledChanged
Fired when the markupEnabled property has changed.
EventObject Type: PropertyChangedEvent<TextView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of markupEnabled. |
textChanged
Fired when the text property has changed.
EventObject Type: PropertyChangedEvent<TextView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of text. |
textColorChanged
Fired when the textColor property has changed.
EventObject Type: PropertyChangedEvent<TextView, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of textColor. |
maxLinesChanged
Fired when the maxLines property has changed.
EventObject Type: PropertyChangedEvent<TextView, number | null>
Property | Type | Description |
---|---|---|
value | number | null |
The new value of maxLines. |
lineSpacingChanged
Fired when the lineSpacing property has changed.
EventObject Type: PropertyChangedEvent<TextView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of lineSpacing. |
fontChanged
Fired when the font property has changed.
EventObject Type: PropertyChangedEvent<TextView, FontValue>
Property | Type | Description |
---|---|---|
value | FontValue |
The new value of font. |