Class “Composite”
Object > NativeObject > Widget > Composite
An empty widget that can contain other widgets.
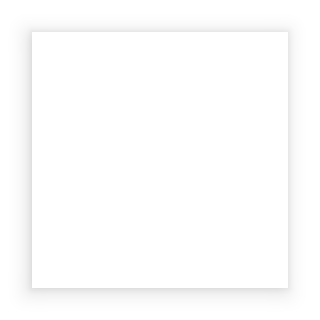
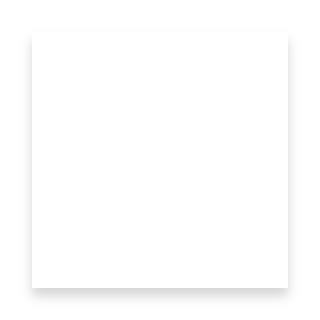
Type: | Composite<ChildType> extends Widget |
Generics: | ChildType: The common widget class of the children this composite can contain. Must be a subclass of Widget and defaults to Widget . |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | Canvas , Cell , CollectionView , ContentView , NavigationView , Page , RefreshComposite , Row , ScrollView , Stack , Tab , TabFolder |
JSX Support: | Element: <Composite/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Any standalone widget element |
Examples
JavaScript
import {Composite, contentView} from 'tabris';
new Composite({left: 0, top: 0, width: 128, height: 256})
.appendTo(contentView);
See also:
JSX Creating a simple Composite
Constructor
new Composite(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<Composite> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Methods
append(…widgets)
Adds the given widgets to the composite.
Parameter | Type | Description |
---|---|---|
…widgets | Widget[] |
Returns: this
append(widgets)
Adds all widgets in the given array to the composite.
Parameter | Type | Description |
---|---|---|
widgets | Widget[] |
Returns: this
append(widgets)
Adds all widgets in the given collection to the composite.
Parameter | Type | Description |
---|---|---|
widgets | WidgetCollection |
Returns: this
apply(options, rules)
Applies the given attributes to all descendants that match the associated selector(s). Each attributes object may contain properties to be set and listeners to be registered. An entry will be treated as a listener if it follows the naming scheme “onEventType”. IMPORTANT: Listeners previously registered (for the same type) via the apply
method, a JSX element attribute or a widget factory call will be de-registered. This means you can call apply repeatedly and have a deterministic outcome. Listeners registered programmatically (e.g. widget.onTap(...)
are not affected by this.)
For better type safety enable strict mode and use the Set
to create properties objects.
If you wish to always exclude specific “internal” children from this, overwrite the children
method on their parent. See “Encapsulation”.
Parameter | Type | Description |
---|---|---|
options | { |
If mode is set to 'strict' the function checks that all selector match at least one widget, and that id selector match exactly one widget. A trigger is string to be associated with the given rulset. If set to 'update' , the ruleset will be applied once immediately and then again every time apply('update') is called. If set to any event-attribute name, such as 'onTap' , it will automatically re-apply the ruleset when this event is triggered. |
rules | {[selector]: Attributes} |
The ruleset to apply. May also be given as a callback which is passed to the widget instance and must return the actual ruleset. This parameter can also be null if the trigger option is set. This will stop re-applying the ruleset previously associated with that trigger. |
Returns: this
apply(rules)
Shorthand for apply({mode: 'default'}, rules)
Parameter | Type | Description |
---|---|---|
rules | {[selector]: Attributes} |
The ruleset to apply. May also be given as a callback which is passed to the widget instance and must return the actual ruleset. |
Returns: this
apply(mode, rules)
Shorthand for apply({mode: mode}, rules})
Parameter | Type | Description |
---|---|---|
mode | 'default' | 'strict' |
If this is set to 'strict' the function checks that all selector match at least one widget, and that id selector match exactly one widget. |
rules | {[selector]: Attributes} |
The ruleset to apply. May also be given as a callback which is passed to the widget instance and must return the actual ruleset. |
Returns: this
apply(trigger)
Applies the ruleset from the last call with trigger
set to 'update'
.
Parameter | Type | Description |
---|---|---|
trigger | 'update' |
Returns: this
children(selector?)
Returns a (possibly empty) collection of all children of this widget that match the given selector.
When writing custom UI components it may be useful to overwrite this method to prevent access to the internal children by external code. Doing so also affects find
and apply
, on this widget as well as on all parents, thereby preventing accidental clashes of widget id or class values. See “Encapsulation”.
Parameter | Type | Description |
---|---|---|
selector | Selector |
A selector expression or a predicate function to filter the results. Optional. |
Returns: WidgetCollection<ChildType>
find(selector?)
Returns a collection containing all descendants of all widgets in this collection that match the given selector.
If you wish to always exclude specific “internal” children from the result, overwrite the children
method (details) to return an empty WidgetCollection
, or use the @component
decorator. These children will then only be visible via the protected _find
method. See “Encapsulation”
Parameter | Type | Description |
---|---|---|
selector | Selector |
A selector expression or a predicate function to filter the results. Optional. |
Returns: WidgetCollection
Protected Methods
These methods are accessible only in classes extending Composite
.
_acceptChild(child)
Called by the framework with each widget that is about to be added as a child of this composite. May be overwritten to reject some or all children by returning false
.
Parameter | Type | Description |
---|---|---|
child | Widget |
Returns: boolean
_addChild(child, index?)
Called by the framework with a child to be assigned to this composite. Triggers the ‘addChild’ event. May be overwritten to run any code prior or after the child is inserted.
Parameter | Type | Description |
---|---|---|
child | Widget |
|
index | number |
Optional. |
Returns: undefined
_apply(options, rules)
Identical to the apply(options, rules)
method, but intended to be used by subclasses in case the children
method was overwritten . See “Encapsulation”
Parameter | Type | Description |
---|---|---|
options | { |
If mode is set to 'strict' the function checks that all selector match at least one widget, and that id selector match exactly one widget. A trigger is string to be associated with the given rulset. If set to 'update' , the ruleset will be applied once immediately and then again every time apply('update') is called. If set to any event-attribute name, such as 'onTap' , it will automatically re-apply the ruleset when this event is triggered. |
rules | {[selector]: Attributes} |
The ruleset to apply. May also be given as a callback which is passed to the widget instance and must return the actual ruleset. |
Returns: this
_apply(rules)
Identical to the apply(rules)
method, but intended to be used by subclasses in case the children
method was overwritten . See “Encapsulation”
Parameter | Type | Description |
---|---|---|
rules | {[selector]: Attributes} |
The ruleset to apply. May also be given as a callback which is passed to the widget instance and must return the actual ruleset. |
Returns: this
_apply(mode, rules)
Identical to the apply(mode, rules)
method, but intended to be used by subclasses in case the children
method was overwritten . See “Encapsulation”
Parameter | Type | Description |
---|---|---|
mode | 'default' | 'strict' |
If this is set to 'strict' the function checks that all selector match at least one widget, and that id selector match exactly one widget. |
rules | {[selector]: Attributes} |
The ruleset to apply. May also be given as a callback which is passed to the widget instance and must return the actual ruleset. |
Returns: this
_apply(trigger)
Applies the ruleset from the last call with trigger
set to 'update'
.
Parameter | Type | Description |
---|---|---|
trigger | 'update' |
Returns: this
_checkLayout(value)
Called by the framework with the layout about to be assigned to this composite. May be overwritten to reject a layout by throwing an Error.
Parameter | Type | Description |
---|---|---|
value | Layout |
Returns: undefined
_children(selector?)
Identical to the children
method, but intended to be used by subclasses in case the children
method was overwritten. See “Encapsulation”.
Parameter | Type | Description |
---|---|---|
selector | Selector |
A selector expression or a predicate function to filter the results. Optional. |
Returns: WidgetCollection
_find(selector?)
Identical to the find
method, but intended to be used only by subclasses (custom components) that encapsulate their children. This is the case if the children
method was overwritten or the @component
decorator is used on the subclass.
Parameter | Type | Description |
---|---|---|
selector | Selector |
A selector expression or a predicate function to filter the results. Optional. |
Returns: WidgetCollection
_initLayout(props?)
Called with the constructor paramter (if any) to initialize the composite’s layout manager. May be overwritten to customize/replace the layout. The new implementation must make a super call to initialize the layout.
Parameter | Type | Description |
---|---|---|
props | { |
Optional. |
Returns: undefined
_removeChild(child)
Called by the framework with a child to be removed from this composite. Triggers the ‘removeChild’ event. May be overwritten to run any code prior or after the child is removed.
Parameter | Type | Description |
---|---|---|
child | Widget |
Returns: undefined
Properties
layout
The layout manager responsible for interpreting the layoutData
of the child widgets of this Composite.
Type: | Layout | null |
Default: | Layout |
Settable: | By Constructor or JSX |
Change Event: | Not supported |
This property can only be set via constructor or JSX. Once set, it cannot change anymore.
Events
addChild
Fired when a child is added to this widget.
EventObject Type: CompositeAddChildEvent<Composite>
Property | Type | Description |
---|---|---|
child | Widget |
The widget that is added as a child. |
index | number |
Denotes the position in the children list at which the child widget is added. |
removeChild
Fired when a child is removed from this widget.
EventObject Type: CompositeRemoveChildEvent<Composite>
Property | Type | Description |
---|---|---|
child | Widget |
The widget that is removed. |
index | number |
The property index denotes the removed child widget’s position in the children list.` |