Class “Video”
Object > NativeObject > Widget > Video
A widget that plays a video from an URL.
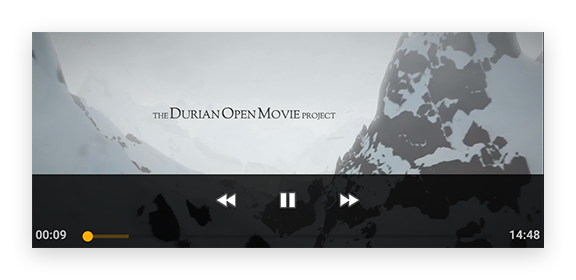
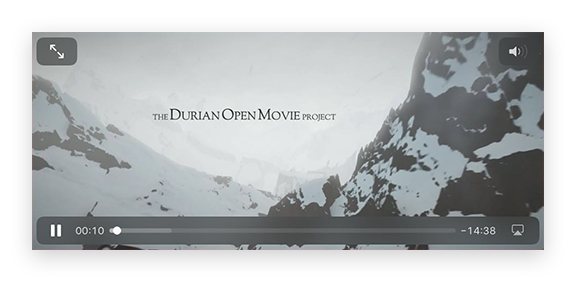
Type: | Video extends Widget |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <Video/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Not Supported |
Examples
JavaScript
import {Video, contentView} from 'tabris';
new Video({
width: 160, height: 90,
url: 'resources/video.mp4'
}).appendTo(contentView);
See also:
Constructor
new Video(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<Video> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Methods
pause()
Pauses the video. state changes to pause
and speed
to 0
. Has no effect when state is not play
.
Returns: undefined
play(speed?)
Starts playing the video, state changes to play
. Has no effect unless the current state is either pause
or ready
.
Parameter | Type | Description |
---|---|---|
speed | number |
Desired playback speed. If the given speed is not supported by the platform or video, the actual playback speed will be 1 - i.e. the natural speed of the video. Optional. |
Returns: undefined
seek(position)
Attempts to change the position
to the given time index. Success depends on the currently loaded video. Has no effect if the current state is empty
or fail
.
Parameter | Type | Description |
---|---|---|
position | number |
Desired position in milliseconds. |
Returns: undefined
Properties
autoPlay
If set to true
, starts playing the video as soon as the state changes from open
to ready
.
Type: | boolean |
Default: | true |
Settable: | Yes |
Change Event: | autoPlayChanged |
controlsVisible
If set to true
, overlays the video with a native UI for controlling playback.
Type: | boolean |
Default: | true |
Settable: | Yes |
Change Event: | controlsVisibleChanged |
duration
Returns the full length of the current video in milliseconds.
Type: | number |
Settable: | No |
Change Event: | durationChanged |
position
Returns the current playback position in milliseconds. This property does not trigger any change events.
Type: | number |
Settable: | No |
Change Event: | positionChanged |
speed
Returns the current playback speed. The value 1
represents the natural speed of the video. When the state of the widget is not play
this property always has the value 0
.
Type: | number |
Settable: | No |
Change Event: | speedChanged |
state
The current video playback state of the widget.
'empty'
- Nourl
has been set.'open'
- Theurl
has been set to a valid value but the widget is not yet ready to play.'ready'
- The widget has loaded enough content to be ready to play, but is not yet playing.'play'
- A video is currently playing.'stale'
- The video is paused because it is buffering more content and will resume playback once it has enough content.'pause'
- Playback is paused because of user input orpause()
has been called.'finish'
- Playback stopped at the end of the video.'fail'
- An error occurred preventing video playback.
Type: | 'empty' | 'open' | 'ready' | 'play' | 'stale' | 'pause' | 'finish' | 'fail' |
Default: | 'empty' |
Settable: | No |
Change Event: | stateChanged |
url
The URL of the video to play. Setting this property to any non-empty string changes the state to open
and the video starts loading. Setting this property to an empty string unloads the current video and the state returns to empty
.
Type: | string |
Settable: | Yes |
Change Event: | urlChanged |
Change Events
urlChanged
Fired when the url property has changed.
EventObject Type: PropertyChangedEvent<Video, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of url. |
controlsVisibleChanged
Fired when the controlsVisible property has changed.
EventObject Type: PropertyChangedEvent<Video, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of controlsVisible. |
autoPlayChanged
Fired when the autoPlay property has changed.
EventObject Type: PropertyChangedEvent<Video, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of autoPlay. |
speedChanged
Fired when the speed property has changed.
EventObject Type: PropertyChangedEvent<Video, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of speed. |
positionChanged
Fired when the position property has changed.
EventObject Type: PropertyChangedEvent<Video, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of position. |
durationChanged
Fired when the duration property has changed.
EventObject Type: PropertyChangedEvent<Video, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of duration. |
stateChanged
Fired when the state property has changed.
EventObject Type: PropertyChangedEvent<Video, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of state. |