Class “Picker”
Object > NativeObject > Widget > Picker
A widget with a drop-down list of items to choose from.
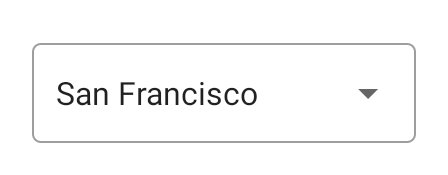
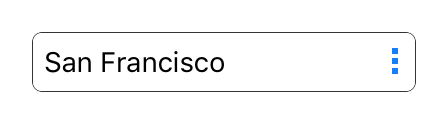
Type: | Picker extends Widget |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | ItemPicker |
JSX Support: | Element: <Picker/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Not Supported |
Examples
JavaScript
import {contentView, Picker} from 'tabris';
const items = ['Apple', 'Banana', 'Cherry'];
new Picker({
itemCount: items.length,
itemText: index => items[index]
}).onSelect(event => console.log(`Selected ${items[event.index]}`))
.appendTo(contentView);
See also:
JSX Creating a simple Picker
JSX picker-style.jsx
Constructor
new Picker(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<Picker> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Properties
borderColor
The color of the Picker border. This can be the surrounding line or the underline of the Picker depending on the style
property.
Type: | ColorValue |
Settable: | Yes |
Change Event: | borderColorChanged |
floatMessage
Android
Whether the hint message should float above the Picker when focus is gained.
Type: | boolean |
Default: | true |
Settable: | Yes |
Change Event: | floatMessageChanged |
font
The font used for the text inside the Picker.
Type: | FontValue |
Settable: | Yes |
Change Event: | fontChanged |
itemCount
The number of items to display.
Type: | number |
Settable: | Yes |
Change Event: | itemCountChanged |
itemText
A function that returns the string to display for a given index.
Type: | (index) => string |
Settable: | Yes |
Change Event: | itemTextChanged |
message
A hint text that is displayed when the picker has no selection.
Type: | string |
Settable: | Yes |
Change Event: | messageChanged |
selectionIndex
The index of the currently selected item.
Type: | number |
Settable: | Yes |
Change Event: | selectionIndexChanged |
style
Android
The visual appearance of the Picker
widget.
With the style
outline, fill or underline the message hint will float above the Picker
on Android. This behavior can be controlled with the property floatMessage
. The style
none will remove any background visualization, allowing to create a custom background.
Type: | 'default' | 'outline' | 'fill' | 'underline' | 'none' |
Default: | 'default' |
Settable: | By Constructor or JSX |
Change Event: | Not supported |
This property can only be set via constructor or JSX. Once set, it cannot change anymore.
See also:
textColor
The color of the text.
Type: | ColorValue |
Settable: | Yes |
Change Event: | textColorChanged |
Events
select
Fired when an item was selected by the user.
EventObject Type: PickerSelectEvent<Picker>
Property | Type | Description |
---|---|---|
index | number |
Contains the index of the selected item. |
Change Events
messageChanged
Fired when the message property has changed.
EventObject Type: PropertyChangedEvent<Picker, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of message. |
floatMessageChanged
Fired when the floatMessage property has changed.
EventObject Type: PropertyChangedEvent<Picker, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of floatMessage. |
itemCountChanged
Fired when the itemCount property has changed.
EventObject Type: PropertyChangedEvent<Picker, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of itemCount. |
itemTextChanged
Fired when the itemText property has changed.
EventObject Type: PropertyChangedEvent<Picker, Function>
Property | Type | Description |
---|---|---|
value | (index) => string |
The new value of itemText. |
selectionIndexChanged
Fired when the selectionIndex property has changed.
EventObject Type: PropertyChangedEvent<Picker, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of selectionIndex. |
borderColorChanged
Fired when the borderColor property has changed.
EventObject Type: PropertyChangedEvent<Picker, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of borderColor. |
textColorChanged
Fired when the textColor property has changed.
EventObject Type: PropertyChangedEvent<Picker, ColorValue>
Property | Type | Description |
---|---|---|
value | ColorValue |
The new value of textColor. |
fontChanged
Fired when the font property has changed.
EventObject Type: PropertyChangedEvent<Picker, FontValue>
Property | Type | Description |
---|---|---|
value | FontValue |
The new value of font. |