Class “DateDialog”
Object > NativeObject > Popup > DateDialog
A DateDialog
represents a native dialog pop-up allowing the user to pick a date. Properties can only be set before open() is called. The dialog is automatically disposed when closed.
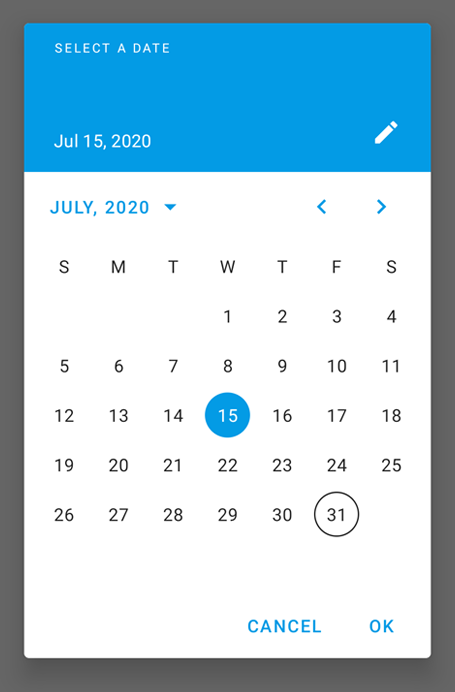
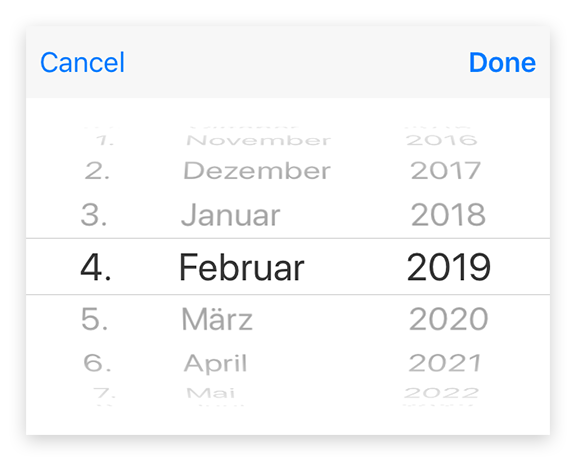
Type: | DateDialog extends Popup |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | None |
JSX Support: | Element: <DateDialog/> Parent Elements: Not supported Child Elements: Not Supported |
Examples
JavaScript
import {DateDialog} from 'tabris';
new DateDialog()
.onSelect(({date}) => console.log(`Selected ${date}`))
.open();
See also:
JSX Creating a simple DateDialog
Constructor
new DateDialog(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<DateDialog> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Static Methods
open(dateDialog)
Makes the given date dialog visible. Meant to be used with inline-JSX. In TypeScript it also casts the given JSX element from any
to an actual DateDialog.
Parameter | Type | Description |
---|---|---|
dateDialog | DateDialog |
The date dialog to open |
Returns: DateDialog
open(date?)
Creates and opens a date dialog.
Parameter | Type | Description |
---|---|---|
date | Date |
The date to be displayed in the dialog. The current date is used when no date is provided. Optional. |
Returns: DateDialog
Properties
date
The date to be displayed in the dialog. The current date is used when no date is provided.
Type: | Date |
Settable: | Yes |
Change Event: | dateChanged |
maxDate
Limits the selectable date range to the given future date. No limit is applied when not set.
Type: | Date |
Settable: | Yes |
Change Event: | maxDateChanged |
minDate
Limits the selectable date range to the given past date. No limit is applied when not set.
Type: | Date |
Settable: | Yes |
Change Event: | minDateChanged |
Events
close
Fired when the date dialog was closed.
EventObject Type: DateDialogCloseEvent<DateDialog>
Property | Type | Description |
---|---|---|
date | Date | null |
The selected date. Can be null when no date was selected. |
select
Fired when a date was selected by the user.
EventObject Type: DateDialogSelectEvent<DateDialog>
Property | Type | Description |
---|---|---|
date | Date |
The selected date. Only the date components reflect the users selection. The time component values are undefined. |
Change Events
dateChanged
Fired when the date property has changed.
EventObject Type: PropertyChangedEvent<DateDialog, Date>
Property | Type | Description |
---|---|---|
value | Date |
The new value of date. |
minDateChanged
Fired when the minDate property has changed.
EventObject Type: PropertyChangedEvent<DateDialog, Date>
Property | Type | Description |
---|---|---|
value | Date |
The new value of minDate. |
maxDateChanged
Fired when the maxDate property has changed.
EventObject Type: PropertyChangedEvent<DateDialog, Date>
Property | Type | Description |
---|---|---|
value | Date |
The new value of maxDate. |