Class “CollectionView”
Object > NativeObject > Widget > Composite > CollectionView
A scrollable list that displays data items in cells, one per row. Cells are created on demand by the createCell callback and reused on scrolling.
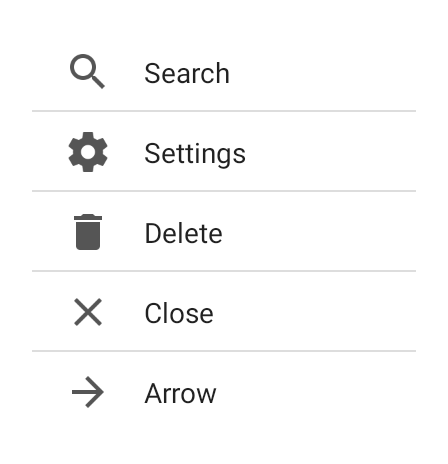
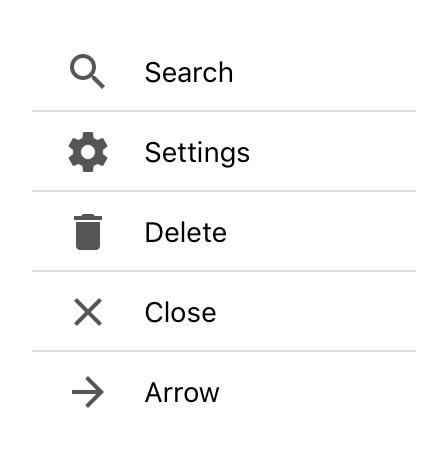
Type: | CollectionView<CellWidgetType> extends Composite<CellWidgetType> |
Generics: | CellWidgetType: The common widget class used for cells in this collectionView. It is recommended to use different classes for different cell types. Must be a subclass of Widget and defaults to Widget . |
Constructor: | public |
Singleton: | No |
Namespace: | tabris |
Direct subclasses: | ListView |
JSX Support: | Element: <CollectionView/> Parent Elements: <Canvas/> , <Cell/> , <Composite/> , <Page/> , <RefreshComposite/> , <Row/> , <ScrollView/> , <Stack/> , <Tab/> Child Elements: Not Supported |
Examples
JavaScript
import {CollectionView, contentView, TextView} from 'tabris';
const items = ['Apple', 'Banana', 'Cherry'];
new CollectionView({
left: 0, top: 0, right: 0, bottom: 0,
itemCount: items.length,
createCell: () => new TextView(),
updateCell: (view, index) => {
view.text = items[index];
}
}).appendTo(contentView);
See also:
JSX Creating a simple CollectionView
JSX Creating a CollectionView with multiple cell types
JSX Creating a CollectionView with pull-to-refresh support
JSX Creating a CollectionView with sticky headers
JSX Creating a CollectionView with dynamic column count
JSX collectionview-cellheightauto.jsx
TSX collectionview-celltype-ts.tsx
TSX collectionview-scroll-ts.tsx
JSX collectionview-swipe-to-dismiss.jsx
TSX collectionview-ts.tsx
Constructor
new CollectionView(properties?)
Parameter | Type | Description |
---|---|---|
properties | Properties<CollectionView> |
Sets all key-value pairs in the properties object as widget properties. Optional. |
Methods
cellByItemIndex(itemIndex)
Returns the cell currently associated with the given item index. Returns null
if the item is not currently displayed.
Parameter | Type | Description |
---|---|---|
itemIndex | number |
The index of the item as given in updateCell . |
Returns: CellWidgetType | null
insert(index, count?)
Inserts one or more items at the given index. When no count is specified, a single item will be added at the given index. New cells may be created if needed. The updateCell
callback will only be called for those new items that become immediately visible. Note that inserting new items changes the index of all subsequent items. This operation will update the itemCount
property.
Parameter | Type | Description |
---|---|---|
index | number |
|
count | number |
The position to insert the items at. A negative index is interpreted as relative to the end. If the given index is greater than the item count, new items will be appended at the end. Optional. |
Returns: undefined
itemIndex(widget)
Determines the item index currently associated with the given cell.
Parameter | Type | Description |
---|---|---|
widget | Widget |
A widget instance created by createCell , or a child of that widget. |
Returns: number
load(itemCount)
Loads a new model with the given itemCount. This operation will update the itemCount
property.
Parameter | Type | Description |
---|---|---|
itemCount | number |
The number of items in the model to load. |
Returns: undefined
refresh(index?)
Triggers an update of the item at the given index by calling the updateCell
callback of the corresponding. If no index is given, all visible items will be updated.
Parameter | Type | Description |
---|---|---|
index | number |
The index of the item that was changed. Optional. |
Returns: undefined
remove(index, count?)
Removes one or more items beginning with the given index. When no count is given, only the item at index will be removed. Note that this changes the index of all subsequent items, however. This operation will update the itemCount
property.
Parameter | Type | Description |
---|---|---|
index | number |
The index of the first item to remove. A negative value is interpreted as relative to the end. |
count | number |
The number of items to remove. Optional. |
Returns: undefined
reveal(index, options?)
Scrolls the item with the given index into view.
Parameter | Type | Description |
---|---|---|
index | number |
The index of the item to reveal. If this is negative, it is interpreted as relative to the end |
options | {animate?: boolean} |
An additional object to control the animation. Set to {animate: false} to scroll without an animation. The animate property defaults to true when no options object is given. Optional. |
Returns: undefined
Properties
cellHeight
The height of a collection cell. If set to 'auto'
, the cell height will be calculated individually for each cell. If set to a function, this function will be called for every item, providing the item index and the cell type as parameters, and must return the cell height for the given item.
Note: On iOS "auto"
may cause significant performance downgrade as it requires additional layouting passes to calculate cell height internally. If possible please use a combination of fixed itemHeight
and cellType
properties to specify different height for different cells.
Type: | number |
Default: | 'auto' |
Settable: | Yes |
Change Event: | cellHeightChanged |
cellType
The name of the cell type to use for the item at the given index. This name will be passed to the createCell
and cellHeight
callbacks. Cells will be reused only for those items that map to the same cell type. If set to a function, this function will be called for every item, providing the item index as a parameter, and must return a unique name for the cell type to use for the given item.
Type: | string |
Settable: | Yes |
Change Event: | cellTypeChanged |
See also:
TSX collectionview-celltype-ts.tsx
JSX collectionview-celltype.jsx
columnCount
The number of columns to display in the collection view. If set to a value n > 1
, each row will contain n
items. The available space will be equally distributed between columns.
Type: | number |
Default: | 1 |
Settable: | Yes |
Change Event: | columnCountChanged |
See also:
JSX collectionview-columncount.jsx
createCell
A callback used to create a new reusable cell widget for a given type. This callback will be called by the framework and the created cell will be reused for different items. The created widget should be populated in the updateCell
function.
Type: | (cellType) => CellWidgetType |
Settable: | Yes |
Change Event: | createCellChanged |
firstVisibleIndex
The index of the first item that is currently visible on screen.
Type: | number |
Settable: | No |
Change Event: | firstVisibleIndexChanged |
itemCount
The number of items to display. To add or remove items later, use the methods insert()
and remove()
instead of setting the itemCount
. To display a new list of items, use the load()
method.
Type: | number |
Settable: | Yes |
Change Event: | itemCountChanged |
lastVisibleIndex
The index of the last item that is currently visible on screen.
Type: | number |
Settable: | No |
Change Event: | lastVisibleIndexChanged |
refreshEnabled
Enables the user to trigger a refresh by using the pull-to-refresh gesture.
Type: | boolean |
Default: | false |
Settable: | Yes |
Change Event: | refreshEnabledChanged |
See also:
JSX collectionview-refreshenabled.jsx
refreshIndicator
Whether the refresh indicator is currently visible. Will be set to true
when a refresh event is triggered. Reset it to false
when the refresh is finished.
Type: | boolean |
Default: | false |
Settable: | Yes |
Change Event: | refreshIndicatorChanged |
refreshMessage
iOS
The message text displayed together with the refresh indicator.
Type: | string |
Default: | (empty string) |
Settable: | Yes |
Change Event: | refreshMessageChanged |
scrollbarVisible
Allows to show or hide the scroll bar. When the scroll bar is hidden, it will be briefly visible while scrolling.
Type: | boolean |
Default: | true |
Settable: | Yes |
Change Event: | scrollbarVisibleChanged |
updateCell
A callback used to update a given cell widget to display the item with the given index. This callback will be called by the framework.
Type: | (cell, index) => void |
Settable: | Yes |
Change Event: | updateCellChanged |
Events
refresh
Fired when the user requested a refresh. An event listener should reset the refreshIndicator property when refresh is finished.
EventObject Type: EventObject<CollectionView>
This event has no additional parameter.
scroll
Fired while the collection view is scrolling.
EventObject Type: CollectionViewScrollEvent<CollectionView>
Property | Type | Description |
---|---|---|
deltaX | number |
Currently always 0. |
deltaY | number |
The delta of the scroll position. Positive when scrolling up and negative when scrolling down. |
See also:
TSX collectionview-scroll-ts.tsx
JSX collectionview-scroll.jsx
Change Events
scrollbarVisibleChanged
Fired when the scrollbarVisible property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of scrollbarVisible. |
cellHeightChanged
Fired when the cellHeight property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, number
| 'auto'
| Function>
Property | Type | Description |
---|---|---|
value | number |
The new value of cellHeight. |
itemCountChanged
Fired when the itemCount property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of itemCount. |
createCellChanged
Fired when the createCell property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, Function>
Property | Type | Description |
---|---|---|
value | (cellType) => CellWidgetType |
The new value of createCell. |
updateCellChanged
Fired when the updateCell property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, Function>
Property | Type | Description |
---|---|---|
value | (cell, index) => void |
The new value of updateCell. |
cellTypeChanged
Fired when the cellType property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, string
| Function
| null>
Property | Type | Description |
---|---|---|
value | string |
The new value of cellType. |
refreshEnabledChanged
Fired when the refreshEnabled property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of refreshEnabled. |
refreshIndicatorChanged
Fired when the refreshIndicator property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, boolean>
Property | Type | Description |
---|---|---|
value | boolean |
The new value of refreshIndicator. |
refreshMessageChanged
Fired when the refreshMessage property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, string>
Property | Type | Description |
---|---|---|
value | string |
The new value of refreshMessage. |
firstVisibleIndexChanged
Fired when the firstVisibleIndex property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of firstVisibleIndex. |
lastVisibleIndexChanged
Fired when the lastVisibleIndex property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of lastVisibleIndex. |
columnCountChanged
Fired when the columnCount property has changed.
EventObject Type: PropertyChangedEvent<CollectionView, number>
Property | Type | Description |
---|---|---|
value | number |
The new value of columnCount. |