Class “Composite”
Object
> NativeObject
> Widget
> Composite
An empty widget that can contain other widgets.
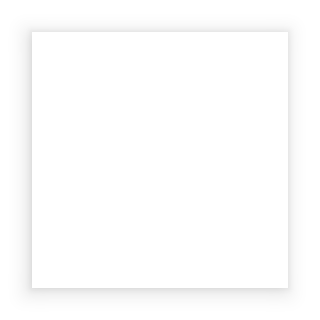
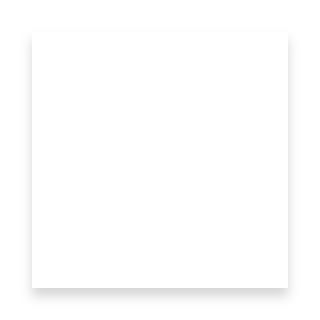
TypeScript type | Composite<ChildType extends Widget = Widget> extends Widget |
Constructor | public |
Singleton | No |
Namespace | tabris |
Direct subclasses | Canvas , ContentView , NavigationView , Page , RefreshComposite , ScrollView , Stack , Tab , TabFolder |
JSX support | Element: <Composite/> Parent element: <Composite/> and any widget extending Composite Child elements: Widgets Text content: Not supported |
Example
import {Composite, contentView} from 'tabris';
new Composite({left: 0, top: 0, width: 128, height: 256})
.appendTo(contentView);
See also:
JSX Creating a simple Composite
Constructor
new Composite(properties?)
Parameter | Type | Optional | Description |
---|---|---|---|
properties | Properties<Composite> |
Yes | Sets all key-value pairs in the properties object as widget properties. |
Methods
append(…widgets)
Adds the given widgets to the composite.
Parameter | Type | Optional | Description |
---|---|---|---|
…widgets | Widget[] |
No |
Returns this
append(widgets)
Adds all widgets in the given array to the composite.
Parameter | Type | Optional | Description |
---|---|---|---|
widgets | Widget[] |
No |
Returns this
append(widgets)
Adds all widgets in the given collection to the composite.
Parameter | Type | Optional | Description |
---|---|---|---|
widgets | WidgetCollection |
No |
Returns this
apply(properties)
Applies the given properties to all descendants that match the associated selector(s).
If you wish to always exclude specific “internal” children from this, overwrite the children
method on their parent. See children
for details.
Parameter | Type | Optional | Description |
---|---|---|---|
properties | object |
No | An object in the format {Selector: {property: value, property: value, ... }, Selector: ...} |
Returns this
children(selector?)
Returns a (possibly empty) collection of all children of this widget that match the given selector.
When writing custom UI components it may be useful to overwrite this method to prevent access to the internal children by external code. Doing so also affects find
and apply
, on this widget as well as on all parents, thereby preventing accidental clashes of widget id or class values. See also _children
, _find
and _apply
.
Parameter | Type | Optional | Description |
---|---|---|---|
selector | Selector |
Yes | A selector expression or a predicate function to filter the results. |
Returns WidgetCollection
find(selector?)
Returns a collection containing all descendants of all widgets in this collection that match the given selector.
If you wish to always exclude specific “internal” children from the result, overwrite the children
method on their parent. See children
for details.
Parameter | Type | Optional | Description |
---|---|---|---|
selector | Selector |
Yes | A selector expression or a predicate function to filter the results. |
Returns WidgetCollection
Protected Methods
These methods are accessible only in classes extending Composite.
_acceptChild(child)
Called by the framework with each widget that is about to be added as a child of this composite. May be overwritten to reject some or all children by returning false
.
Parameter | Type | Optional | Description |
---|---|---|---|
child | Widget |
No |
Returns boolean
_addChild(child, index?)
Called by the framework with a child to be assigned to this composite. Triggers the ‘addChild’ event. May be overwritten to run any code prior or after the child is inserted.
Parameter | Type | Optional | Description |
---|---|---|---|
child | Widget |
No | |
index | number |
Yes |
Returns void
_apply(properties)
Identical to the apply
method, but intended to be used by subclasses in case the children
method was overwritten . See children
for details.
Parameter | Type | Optional | Description |
---|---|---|---|
properties | object |
No | An object in the format {Selector: {property: value, property: value, ... }, Selector: ...} |
Returns this
_checkLayout(value)
Called by the framework with the layout about to be assigned to this composite. May be overwritten to reject a layout by throwing an Error.
Parameter | Type | Optional | Description |
---|---|---|---|
value | Layout |
No |
Returns void
_children(selector?)
Identical to the children
method, but intended to be used by subclasses in case the children
method was overwritten. See children
for details.
Parameter | Type | Optional | Description |
---|---|---|---|
selector | Selector |
Yes | A selector expression or a predicate function to filter the results. |
Returns WidgetCollection
_find(selector?)
Identical to the find
method, but intended to be used by subclasses in case the children
method was overwritten. See children
for details.
Parameter | Type | Optional | Description |
---|---|---|---|
selector | Selector |
Yes | A selector expression or a predicate function to filter the results. |
Returns WidgetCollection
_initLayout(props?)
Called with the constructor paramter (if any) to initialize the composite’s layout manager. May be overwritten to customize/replace the layout. The new implementation must make a super call to initialize the layout.
Parameter | Type | Optional | Description |
---|---|---|---|
props | {layout?: Layout} |
Yes |
Returns void
_removeChild(child)
Called by the framework with a child to be removed from this composite. Triggers the ‘removeChild’ event. May be overwritten to run any code prior or after the child is removed.
Parameter | Type | Optional | Description |
---|---|---|---|
child | Widget |
No |
Returns void
Properties
layout
The layout manager responsible for interpreting the layoutData
of the child widgets of this Composite.
Type | Layout | null |
Default | Layout |
Settable | On creation |
Change events | No |
This property can only be set via constructor or JSX. Once set, it cannot change anymore.
Events
addChild
Fired when a child is added to this widget.
Parameter | Type | Description |
---|---|---|
child | Widget |
The widget that is added as a child. |
index | number |
Denotes the position in the children list at which the child widget is added. |
removeChild
Fired when a child is removed from this widget.
Parameter | Type | Description |
---|---|---|
child | Widget |
The widget that is removed. |
index | number |
The property index denotes the removed child widget’s position in the children list.` |