Class “RadioButton”
Object
> NativeObject
> Widget
> RadioButton
A radio button. Selecting a radio button de-selects all its siblings (i.e. all radio buttons within the same parent).
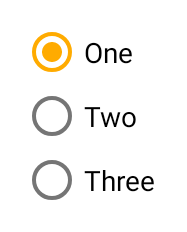
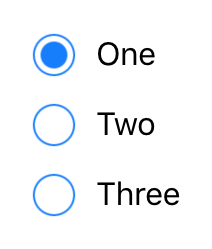
Constructor | public |
Singleton | No |
Namespace | tabris |
Direct subclasses | None |
JSX support | Element: <RadioButton/> Parent element: <Composite/> and any widget extending Composite Child elements: None Text content: Sets text property |
Example
import {RadioButton, contentView} from 'tabris';
new RadioButton({text: 'One', checked: true})
.onSelect(event => console.log(`Checked: ${event.checked}`))
.appendTo(contentView);
new RadioButton({text: 'Two'})
.onSelect(event => console.log(`Checked: ${event.checked}`))
.appendTo(contentView);
See also:
JSX Creating a set of RadioButtons
Constructor
new RadioButton(properties?)
Parameter | Type | Optional | Description |
---|---|---|---|
properties | Properties<RadioButton> |
Yes | Sets all key-value pairs in the properties object as widget properties. |
Properties
checked
The checked state of the radio button.
Type | boolean |
Default | false |
Settable | Yes |
Change events | Yes |
checkedTintColor
The color of the selectable area in checked state. Will fall back to tintColor
if not set.
Type | ColorValue |
Settable | Yes |
Change events | Yes |
font
The font used for the text.
Type | FontValue |
Settable | Yes |
Change events | Yes |
text
The label text of the radio button.
Type | string |
Settable | Yes |
Change events | Yes |
JSX content type | string |
When using RadioButton as an JSX element the element content is mapped to this property. Therefore
<RadioButton>Hello World</RadioButton>
has the same effect as:
<RadioButton text='Hello World' />
textColor
The color of the text.
Type | ColorValue |
Settable | Yes |
Change events | Yes |
tintColor
The color of the selectable area.
Type | ColorValue |
Settable | Yes |
Change events | Yes |
Events
select
Fired when the radio button is selected or deselected by the user.
Parameter | Type | Description |
---|---|---|
checked | boolean |
The new value of checked. |
Change Events
checkedChanged
Fired when the checked property has changed.
Parameter | Type | Description |
---|---|---|
value | boolean |
The new value of checked. |
textChanged
Fired when the text property has changed.
Parameter | Type | Description |
---|---|---|
value | string |
The new value of text. |
textColorChanged
Fired when the textColor property has changed.
Parameter | Type | Description |
---|---|---|
value | ColorValue |
The new value of textColor. |
tintColorChanged
Fired when the tintColor property has changed.
Parameter | Type | Description |
---|---|---|
value | ColorValue |
The new value of tintColor. |
checkedTintColorChanged
Fired when the checkedTintColor property has changed.
Parameter | Type | Description |
---|---|---|
value | ColorValue |
The new value of checkedTintColor. |
fontChanged
Fired when the font property has changed.
Parameter | Type | Description |
---|---|---|
value | FontValue |
The new value of font. |